I've written about creating Conway's Game Of Life in a number of other articles, so I didn't think this was worth a full article.
Here is the code in full.
extends Node2D
# Set up some variables.
var grid = [];
var xSizeFactor = 1;
var ySizeFactor = 1;
var xResolution = 100;
var yResolution = 100;
# Called when the node enters the scene tree for the first time.
func _ready():
xSizeFactor = get_viewport().size.x / xResolution;
ySizeFactor = get_viewport().size.y / yResolution;
createGrid(xResolution, yResolution);
# Called every frame. 'delta' is the elapsed time since the previous frame.
func _process(delta):
grid = runGeneration(xResolution + delta, yResolution + delta);
queue_redraw();
pass;
# Called when CanvasItem has been requested to redraw.
func _draw():
generateBoard(xResolution, yResolution)
pass
# Set up a random grid.
func createGrid(x_resolution, y_resolution):
grid = range(0, x_resolution)
for x in range(0, x_resolution):
grid[x] = range(0, y_resolution)
for y in range(0, y_resolution):
grid[x][y] = randi_range(0, 1)
# Draw a square on the game board for every live cell in the grid.
func generateBoard(x_resolution, y_resolution):
for x in range(0, x_resolution):
for y in range(0, y_resolution):
var realx = x * xSizeFactor
var realy = y * ySizeFactor
if grid[x][y] == 1:
draw_rect(Rect2(realx, realy, xSizeFactor, ySizeFactor), Color(1, 1, 1))
# Run the generation from one grid to another.
func runGeneration(x_resolution, y_resolution):
# Generate new empty grid to populate with result of generation.
var return_grid = range(0, x_resolution)
for x in range(0, x_resolution):
return_grid[x] = range(0, y_resolution)
for y in range(0, y_resolution):
return_grid[x][y] = 0
# Iterate over the grid.
for x in range(0, x_resolution):
for y in range(0, y_resolution):
var neighbours = numberNeighbours(x, y)
if grid[x][y] == 1:
# Current cell is alive.
if neighbours < 2:
# Cell dies (rule 1).
return_grid[x][y] = 0
elif neighbours == 2 or neighbours == 3:
# Cell lives (rule 2).
return_grid[x][y] = 1
elif neighbours > 3:
# Cell dies (rule 3).
return_grid[x][y] = 0
else:
# Current cell is dead.
if neighbours == 3:
# Make cell live (rule 4).
return_grid[x][y] = 1
return return_grid
# Count the number of neighbours.
func numberNeighbours(x, y):
var count = 0
var xrange = [x-1, x, x+1]
var yrange = [y-1, y, y+1]
for x1 in xrange:
for y1 in yrange:
if x1 == x and y1 == y:
# Don't count this cell.
continue
if x1 < grid.size() - 1 and y1 < grid[x1].size() - 1:
# Cell exists.
if grid[x1][y1] == 1:
count += 1
return count
This is all running on a single Node2D scene. If you run the project in debug mode you should see something like this.
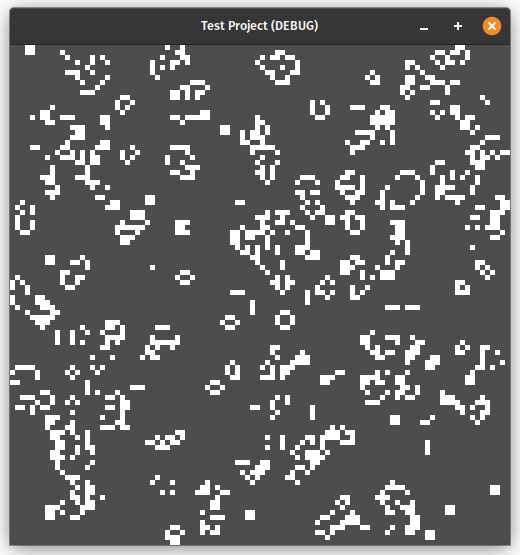
This is in a 500x500 window.
If you want to know more about the Game of Life algorithm then look at the following articles.
Add new comment