When I found about the ability to create QR codes using the Google Charts API I decided to sit down and create a little tool that would generate QR codes for me. I've had this tool for a while and I recently noticed that the QR code mechanism has become deprecated. The API will still work for a couple of years so I thought it was worth posting this. Creating a static QR code using Google Charts is quite easy, all you need to do is create an image. For instance, to create a QR code for the #! code address I would do the following.
<img src="https://chart.googleapis.com/chart?chs=177x177&cht=qr&chl=http://www.hashbangcode.com/&choe=UTF-8" />
Which creates the following image.
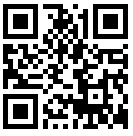
To add a little bit of automation to this I created a HTML page with a textarea and an image. This forms the basis of the tool that will create QR codes.
<textarea cols="100" id="inputtext"></textarea>
<img id="qrcode" src="" />
To keep things easy I decided to use jQuery to interact with the elements on the page, so I created an include for that using another Google service for including JavaScript frameworks called Google Ajax. I then wrote a little function that would update the source of the image every time text was entered into the textarea.
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$('textarea#inputtext').keydown(function(e) {
$('img#qrcode').attr('src', 'https://chart.googleapis.com/chart?chs=177x177&cht=qr&chl=' + $('textarea#inputtext').val() + '&choe=UTF-8');
});
});
</script>
This code works, but if you are tying in anything into the textarea a lot of requests are made to Google and the image is updated lots of times. To stop this I introduced a small timeout so that the image update function would only be run every second, rather than immediately.
<script type="text/javascript">
$(document).ready(function(){
var timeout;
$('textarea#inputtext').keydown(function(e) {
if (timeout) {
window.clearTimeout(timeout);
}
timeout = window.setTimeout(function() {
$('img#qrcode').attr('src', 'https://chart.googleapis.com/chart?chs=177x177&cht=qr&chl=' + $('textarea#inputtext').val() + '&choe=UTF-8');
}, 1000);
});
});
</script>
After this, I extended the tool to allow for the size of the QR code to be changed. This involved adding a simple select element to the form so that I now had the following.
<textarea cols="100" id="inputtext"></textarea>
<select id="qrsize"><option value="29">29</option><option value="57">57</option><option selected="selected" value="177">177</option><option value="500">500</option>
</select>
<img id="qrcode" src="" />
I then incorporated this select element into the jQuery so that the size is incorporated into the QR code call. I also added a clause that runs the keydown() event on the textarea so that the QR code gets updated when a new size is selected.
<script type="text/javascript">
$(document).ready(function(){
var timeout;
$('textarea#inputtext').keydown(function(e) {
if (timeout) {
window.clearTimeout(timeout);
}
timeout = window.setTimeout(function() {
var qrsize = $('select#qrsize').val();
$('img#qrcode').attr('src', 'https://chart.googleapis.com/chart?chs=' + qrsize + 'x' + qrsize + '&cht=qr&chl=' + $('textarea#inputtext').val() + '&choe=UTF-8');
}, 1000);
});
$('select#qrsize').change(function(e) {
$('textarea#inputtext').keydown();
});
});
</script>
If you want to view the tool in action have a look at the QR Code Generator Tool.
Comments
Submitted by esteban briceño on Thu, 10/12/2017 - 15:53
PermalinkHii,
Seriously this post was damn awesome, one thing that strikes me the most is your style of writing… Whoa you explain stuffs so elegantly, I could understand the concept behind this very well just by reading it once, very well written.
Thanks for sharing this wonderful Article.
Submitted by kevin Espanol on Wed, 09/13/2023 - 11:49
PermalinkGreat article! The integration with the Google Charts API makes QR code creation seamless. For those looking for a free QR code generator with more customization options, there are plenty of user-friendly tools available online to explore and try out.
Submitted by Linu on Fri, 04/04/2025 - 07:14
PermalinkAdd new comment