Tying together different select elements in a form is done with very little effort thanks to the ajax and states system built into Drupal 9. This means that any Drupal form can have a select element that shows and updates options into another select element within the same form. Using this system you can create a hierarchical system where one select will show and populate another select element with items dependent on the first. This is great for giving the user the ability to drill down into options that are dependent on each other. As the user selects the first select element the second select element will populate with data and be shown on the screen.
It does, however, require a few things to be in place first and so takes a little while to set up. There are also two different ways to set up this kind of system, both of which have their limitations. I found a lot of information out there on how to implement one system, but not the other. I thought I would create a post to show how each system can be set up and where it can be used.
The Form
Before jumping into the ajax components we need a form. The simplest, independent and most universally accepted set of select elements I could think of is a date picker. You wouldn't normally display the date as a set of select elements (although I have seen this before), but the data behind it is simple enough to understand and doesn't require any other aspects from Drupal to use.
Here is the form we will generate here.
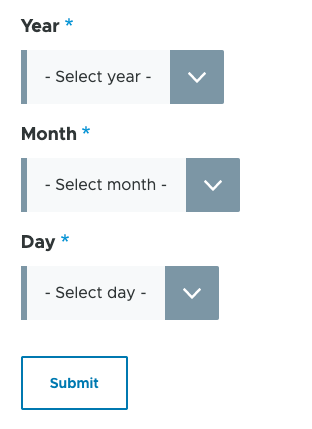
In this state the user can technically select the year, month and day, but because the form is static the number of days will always be the same. If we implement this using Drupal's ajax form update mechanisms we can show the correct number of days depending on the month selected. This also allows for things like leap years to be taken into account so that the number of days in February can be dynamically altered.
Here is the form class in its entirety. We'll be adding to this code throughout the rest of this post. The submit handler just prints out the entered data to the screen, and is a simple way of showing the form in action.
<?php
namespace Drupal\mymodule\Form;
use Drupal\Core\Form\FormBase;
use Drupal\Core\Form\FormStateInterface;
class SelectAjaxDemo extends FormBase {
/**
* {@inheritdoc}
*/
public function getFormId() {
return 'select_ajax_demo';
}
/**
* {@inheritdoc}
*/
public function buildForm(array $form, FormStateInterface $form_state) {
$years = range(2019, 2050);
$years = array_combine($years, $years);
$year = $form_state->getValue('year');
$form['year'] = [
'#type' => 'select',
'#title' => $this->t('Year'),
'#options' => $years,
'#empty_option' => $this->t('- Select year -'),
'#default_value' => $year,
'#required' => TRUE,
];
$months = [1 => 'Jan', 2 => 'Feb', 3 => 'Mar', 4 => 'Apr', 5 => 'May', 6 => 'Jun', 7 => 'Jul', 8 => 'Aug', 9 => 'Sep', 10 => 'Oct', 11 => 'Nov', 12 => 'Dec'];
$month = $form_state->getValue('month');
$form['month'] = [
'#type' => 'select',
'#title' => $this->t('Month'),
'#options' => $months,
'#empty_option' => $this->t('- Select month -'),
'#default_value' => $month,
'#required' => TRUE,
];
$days = range(1, 31);
$day = $form_state->getValue('day');
$form['day'] = [
'#type' => 'select',
'#title' => $this->t('Day'),
'#options' => $days,
'#empty_option' => $this->t('- Select day -'),
'#default_value' => $day,
'#required' => TRUE,
];
$form['submit'] = [
'#type' => 'submit',
'#value' => 'Submit',
];
return $form;
}
/**
* {@inheritdoc}
*/
public function submitForm(array &$form, FormStateInterface $form_state) {
$year = $form_state->getValue('year');
$month = $form_state->getValue('month');
$day = $form_state->getValue('day');
$messenger = \Drupal::messenger();
$messenger->addMessage("Year:" . $year . " Month:" . $month . " Day:" . $day);
}
}
Method One - Single Select Callback
The first method to discuss the simplest to implement and involves just tying each select element to an ajax callback to the form.
The first ajax implementation we need is on the year select element. Setting the ajax event attribute to change means that when the select element is changed the ajax callback is triggered and the month element is changed. The callback attribute is the function we will call in this function and the wrapper is the element that will be replaced.
$form['year'] = [
'#type' => 'select',
'#title' => $this->t('Year'),
'#options' => $years,
'#empty_option' => $this->t('- Select year -'),
'#default_value' => $year,
'#required' => TRUE,
'#ajax' => [
'event' => 'change',
'callback' => '::yearSelectCallback',
'wrapper' => 'field-month',
],
];
Before adding the ajax callback we first need to add a couple of items to the month select element so that it can react correctly to the callback. Because we do not want the month select element to display before the year has been selected we first add a states attribute. This is set so that the current element is not visible until the year select element has something set in it. This isn't technically part of the ajax callback, but creates a nicer user experience with this kind of hierarchical form.
To get the ajax callback to work we set a prefix and suffix on the month form element to wrap the element in a div. This allows us to replace the whole field by simply returning the field from the ajax callback.
$form['month'] = [
'#type' => 'select',
'#title' => $this->t('Month'),
'#options' => $months,
'#empty_option' => $this->t('- Select month -'),
'#default_value' => $month,
'#required' => TRUE,
'#states' => [
'!visible' => [
':input[name="year"]' => ['value' => ''],
],
],
'#prefix' => '<div id="field-month">',
'#suffix' => '</div>',
];
Finally, we set the ajax callback for the years field. The years field callback will return the month field and replace field-month div with the contents returned in the callback.
public function yearSelectCallback(array $form, FormStateInterface $form_state) {
return $form['month'];
}
Here are the sequence of events called when the years select is changed.
- The select element is changed.
- The states API is triggered, and because the year select contains data the month select will be displayed.
- The ajax callback is triggered.
- After bootstrapping Drupal, the entire form() method is run again, generating the form elements.
- The yearSelectCallback() method is called, returning just the month form element.
- Drupal renders the element returned so that it is in HTML.
- The ajax success function (on the JavaScript side) replaces the field-month div with the contents of the returned HTML from Drupal.
Taking this a step further we can now update the day field to do the same.
In order to do this we need to add an ajax callback to the month field. This callback will be triggered by a change to the month select and will call the monthSelectCallback() method in the form class.
$form['month'] = [
'#type' => 'select',
'#title' => $this->t('Month'),
'#options' => $months,
'#empty_option' => $this->t('- Select month -'),
'#default_value' => $month,
'#required' => TRUE,
'#states' => [
'!visible' => [
':input[name="year"]' => ['value' => ''],
],
],
'#ajax' => [
'event' => 'change',
'callback' => '::monthSelectCallback',
'wrapper' => 'field-day',
],
'#prefix' => '<div id="field-month">',
'#suffix' => '</div>',
];
The day field can now be updated in a couple of different ways, and this is where the power of ajax callbacks starts to become really clear. Month and years select elements will contain the same number of items but not every month has the same number of days[citation needed]. This means that when we select a year and a month combination the number of days in the month might change for February in the case of leap years. This means that we can dynamically take in the month and year values and update the select list with the right number of items that represent the day.
The day field is updated with the same states, prefix and suffix attributes that allow the day field to be displayed by the states API and then replaced by the ajax callback.
// Extract the year and month values from the user input (this is already part of the form, but duplicated here for clarity).
$year = $form_state->getValue('year');
$month = $form_state->getValue('month');
// Calculate the number of days in the month.
$number = cal_days_in_month(CAL_GREGORIAN, $month, $year);
$days = range(1, $number);
$day = $form_state->getValue('day');
$form['day'] = [
'#type' => 'select',
'#title' => $this->t('Day'),
'#options' => $days,
'#empty_option' => $this->t('- Select day -'),
'#default_value' => $day,
'#required' => TRUE,
'#states' => [
'!visible' => [
':input[name="month"]' => ['value' => ''],
],
],
'#prefix' => '<div id="field-day">',
'#suffix' => '</div>',
];
The monthSelectCallback() method is pretty simple. All we need to do is return the day field.
public function monthSelectCallback(array $form, FormStateInterface $form_state) {
return $form['day'];
}
This all works nicely. When a user selects the year the month field is shown and when the month is selected the day field (containing the correct number of days) is shown.
One slight problem here is that if the user then selects a different year then the day field isn't updated. This is because the ajax callback for days is not triggered, it is only triggered when the month select element is changed. This is a slight limitation of this method and fixing this requires a slightly different approach, which leads us onto method two.
Method Two - The ReplaceCommand
As the simple callback method has the limitation of not updating the entire form a slight alteration needs to be made. The change will mean that every time a select element on the form is changed all of the elements will be updated with up to date values. This method is slightly more difficult to implement due to the inclusion of a couple of different classes.
The first thing we need to do is change the ajax callbacks to all point at the same method. I've removed some of the detail from the above form setup to reduce the amount of code here as all I am doing is changing the ajax callback attribute.
$form['year'] = [
// ..
'#ajax' => [
'event' => 'change',
'callback' => '::formSelectCallback',
'wrapper' => 'field-month',
],
];
$form['month'] = [
// ..
'#ajax' => [
'event' => 'change',
'callback' => '::formSelectCallback',
'wrapper' => 'field-day',
],
// ..
];
Next we need to add two additional use statements to the top of the form class to introduce the AjaxResponse and ReplaceCommand classes to our form.
use Drupal\Core\Ajax\AjaxResponse;
use Drupal\Core\Ajax\ReplaceCommand;
The single callback function, rather than just returning the form element, needs to return an AjaxResponse object. This object is used by Drupal to run a series of commands, and we use the object to inject a couple of ReplaceCommand objects to replace the form fields. The ReplaceCommand will take an HTML element name and a renderable Drupal item (or just plain HTML) and will replace the element with the supplied HTML. This will run a bunch of JavaScript code without us actually needing to write any JavaScript.
public function formSelectCallback(array $form, FormStateInterface $form_state) {
$response = new AjaxResponse();
$response->addCommand(new ReplaceCommand('#field-month', $form['month']));
$response->addCommand(new ReplaceCommand('#field-day', $form['day']));
return $response;
}
The final output of this is that the form will act in the same way as the simple method with one major difference. When the year field is updated both the method and the day fields are updated at the same time, the upshot of this is that if the year is not a leap year and February is selected as the month then the number of days is automatically updated. The states attributes still hides the month and day fields until they have values in them.
To illustrate this, here is what the form looks like at each step.
Step 1 - The form without any user interaction.
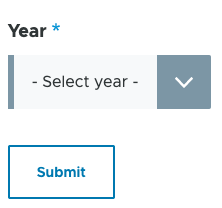
Step 2- The form after selecting the year.
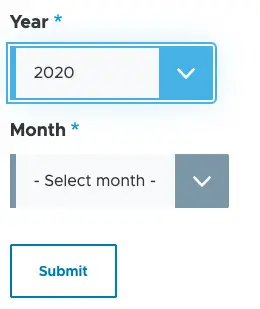
Step 3 - The form after selecting a month.
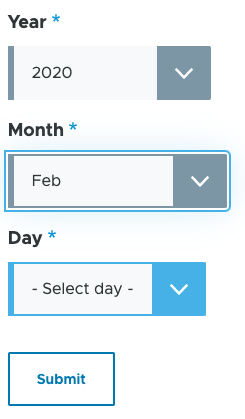
The downside to this method is that when any of the form fields are changed then more of the form is rendered and sent back through the ajax request. This can mean that on large forms there might be a slowdown as everything is reconstructed onto the page through the ajax callback. It might, however, be better to approach forms with a single callback in mind, and then replace all elements at once as it does create a better user experience. You get more control over what is going on within the form as the user fills things in.
Comments
Great and helpful article! Is it possible to make something like this in Entity Forms?
Submitted by Robert on Mon, 02/15/2021 - 09:05
PermalinkThanks Robert! Glad you found it useful.
By Entity Forms, do you mean using hook_form_alter() to inject this sort of thing into a node (or other entity) form? I think that's certainly possible, I think the callback used would just be a stand alone callback function though (ie, not part of a form class).
Submitted by philipnorton42 on Mon, 02/15/2021 - 09:23
PermalinkDon't you getting any validation errors on the second approach as all the fields are marked as required? As you wrote, on every AJAX call the form will be processed and validated as well. I'm using Business Rules module to make dependent fields on entity forms and it seems using the same approach with AJAX command to replace the dependant field. I'm facing the issue when there is three or more levels of dependent fields and they are required. During AJAX call on top level changes the dependent fields has empty values and the form validation fails.
Do you have any suggestions how it could be solved? Maybe fields validation could be skipped only on AJAX calls?
Submitted by Andrew on Tue, 02/23/2021 - 16:02
PermalinkI think you need to look at the "limit_validation_errors" form attribute. That will prevent validation errors from happening and mucking up your forms during ajax calls.
Submitted by philipnorton42 on Thu, 02/25/2021 - 08:33
PermalinkI ve done something like this but with region-> départment-> commune
But my problem is and i m stuck for few days now. If i sleect region the departments are well loaded in select list but when i select department i have error: An unrecoverable error occurred. The uploaded file likely exceeded the maximum file size (32 MB) that this server supports. Do you know what cause this error ?
Submitted by Algas on Mon, 04/11/2022 - 17:47
Permalinki have this error when i select the month : An unrecoverable error occurred. The uploaded file likely exceeded the maximum file size (2 MB) that this server support
Submitted by Algas on Mon, 04/11/2022 - 19:05
PermalinkHi Algas, Thanks for the comment.
I'm only guessing, but it sounds like the Apache suhosin module is causing you some grief?
Submitted by philipnorton42 on Mon, 04/11/2022 - 20:00
PermalinkI just ran into the infamous "maximum file size" bug when attempting to Ajaxify a Views exposed filter form field with Drupal 9.5.3.
This issue dates back to 2017 and development to resolve it is still on-going: https://www.drupal.org/project/drupal/issues/2842525
Submitted by John H on Thu, 02/23/2023 - 23:06
PermalinkCase:
An error is displayed - The submitted value 28 in the Day element is not allowed.
How to avoid this type of error?
Submitted by imibo on Mon, 03/31/2025 - 10:07
PermalinkThe month of February in 2022 only has 28 days, which is probably where the error is coming from. I would suggest clearing out the select element every time you update it and look into using "limit_validation_errors" to suppress errors like that.
Submitted by philipnorton42 on Mon, 03/31/2025 - 21:33
PermalinkAdd new comment