Sunday
Due to the crappy train service from my home town I had to drive into Manchester on Sunday, but made it in good time to have a coffee before we went to vote on the talks. Some people had a heavy night drinking the night before and therefore didn't make it in the morning (or at all). Some has also opted to only attend the Saturday and so there were a few faces missing, but there were still plenty of people there.
JavaScript for PHP Developers
Kathryn Reeve
This talk looked at some common mistakes that PHP developers tend to make when they code in JavaScript. I have seen this talk before at a PHPNW meetup about a year ago, but it was good to sit and hear it again as I remember it as being very content heavy. Kat is an able speaker who has a good knowledge of the subject and clearly speaks from experience.
Variables in JavaScript are mostly the same as PHP in that they are loosely typed. The three different types of JavaScript functions available are Standard, lambda (or those stored in variables) and self executing. Most of the control structures are the same as the PHP variants, there is even a foreach equivalent which has the following syntax.
for (var x in y)
However, this should be avoided unless you really know what you want as it will alter objects in place. This means that the value of y is a reference to the item in x being referenced so changing anything in the loop will change it permanently. Something to be weary of is when you pass system objects into the loop as these will also be altered.
Arrays in JavaScript have numerical indexed only, accessed and created in the same way as PHP, there are no associated arrays. An alternative is to use the "new Array()" object, although this can cause issues as you are using the native array object which might get altered and also has some properties of it's own. It is important in JavaScript to always finish arrays correctly, in PHP you can get away with adding a comma to the end of an array definition without any side effects, but certain browsers will error completely if you do this in JavaScript.
Scoping can be a real problem for PHP devs who are familiar with creating variables on the fly. In JavaScript, there are two possible ways to create a variable, these are with the var keyword and without.
var MyVariable = 1;
MyVariable = 1;
Leaving the var keyword out can be dangerous as JavaScript will treat this variable as a global. Unless you really know that you want a global variable and you know that your variable won't be used by other code then this is fine. What happens when you create a global variable is that JavaScript will look up the object tree to see if the original object was defined. If it is found then that is used instead of your variable so you can create some nasty problems.
The 'this' variable refers to the object you are in. So if you are inside a function this will be the function object. The value of this can be changed using .call() and .apply(), certain frameworks like jQuery do this a lot.
An important part of understanding JavaScript is understanding the Document Object Model (DOM). Everything in the DOM is a child of the window object and children of the DOM are either HTML or text nodes. Finding elements in the DOM is done using a variety of different methods.
var element = document.getElementById('name'); // All browser support
var element = document.getElementsByClassName('class'); // Not ie below 9
var element = document.getElementsByTagName('p'); // All browser support
var element = document.querySelectorAll('p#id'); // Relatively new, all frameworks will pass to this if it is present
Creating elements is done in a two step process by creating the element itself then adding it to the DOM. Creating elements is done using either document.createElement() or document.createTextNode() and adding to the DOM is then done using methods like appendChild() and insertBefore().
On tip that Kat gave was not to add to the DOM during a loop as every time a node is added to the DOM it is rebuilt and skinned, which can cause a large delay if you end up adding 100 items. The best thing to do here is to create a parent node and then add to that element during the loop, only adding the parent node to the DOM when the loop has finished.
JSON is a notation style that allows for objects be created in a more convenient way. It is generally used over AJAX communications as it is easier to parse and smaller to package data in JSON notation.
var obj = {
'msg' : 'a message'
}
Keys should be declared in string format or there is a danger that you will clash with JavaScript keywords. It is possible, when getting a response from an AJAX request to use eval() to convert it into useable data. This should be avoided at all costs as it is a major security risk. To get JavaScript to read JSON you just have to transmit it with the "application/json" header. Most browsers will natively support JSON, but some don't and it is possible to provide support for them by using the json2.js library from Douglas Crockford.
HTML5 And Friends
Patrick H. Lauke
This talk from Patrick was a fantastic introduction to HTML5, starting at the creation of HTML4.01, the movement to XHTML1.0 and then the inception of the new HTML5 standard. It was quite a large topic, but it boiled down to why HTML5 exists and if any developers (or designers) should be adopting it for their website builds.
In 2004 the focus was on getting the XHTML2.0 standard complete, but it was decided that this was too much of a change from HTML4.01 and it would break a lot of sites. So the Web Hypertext Application Technology Working Group (WHATWG) started to create the HTML5 specification and dropped the XHTML 2.0 standard.
The main thing to realise about HTML5 is that it is still a work in progress and not a finished web standard. The finished standard should be ratified in May this year so there isn't long to wait. Some designers feel that it's a little bit too early to be adopting the standard, especially as some interoperability issues have not been fully ratified. However, lots of things work and they work enough that only small changes to browsers will be needed when the standard is published. One thing to realise about HTML5 is that it has different meanings for different people, it is both a marketing term and a specification, which means that it probably gets more attention than other technologies.
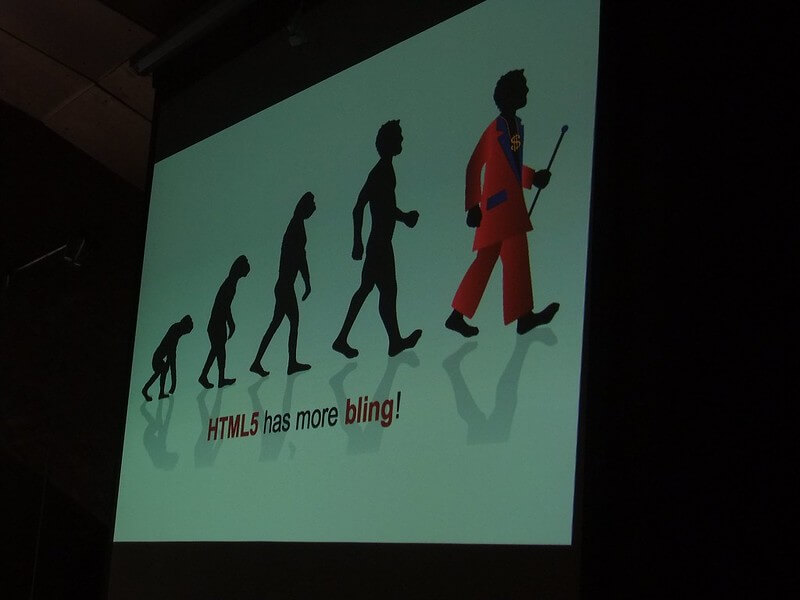
Picture taken by Lorna Mitchell.
HTML5 can be described as HTML4.01 with bling. The spec was specifically written with browser developers in mind in order to avoid the different browser rendering problems from previous years. The thing about the HTML4.01 spec was that it was written with designers in mind and so was interpreted very differently between different vendors, causing lots of issues. Another document has been produced that goes over what a designer needs to know about the HTML5 spec and is updated as and when the full spec is updated.
In terms of syntax and semantics HTML5 tries to standardise but does not aim for purity like XHTML did. Instead it takes a more pragmatic approach and allows for older sites with broken tags and things to work. There are a lot of sites out there and new browsers should be able to render them in the same way as they have always been.
The main problem with the original HTML was the doctype declaration. This has always been copied from one doc to another in what described as "cargo cult code", which I thought was a great term. The main problem was that the browser doesn't really care about doctype, the only thing that complained if the doctype wasn't present was the HTML validator (with the possible exception of quirks mode). The full HTML5 doctype is now as follows, which is much more memorable.
<!DOCTYPE html>
Multiple coding styles are also valid in HTML5, using self closing tags, double and single quotes for attributes and upper and lower case for tags is fine and allowed. Other tags have been simplified so that they don't contain information that isn't needed. A good example of this is the <script></script> and <style></style> tags, the type attribute is no longer needed and it is assumed that JavaScript or CSS are being used.
In fact, the minimal, validated HTML5 document possible is as follows (notice the absence of head and body tags):
<!DOCTYPE htmL><title>Minimal HTML5 document</title>
Obviously a few more elements are needed to build a page and there are several new elements that define the scaffolding of a page. Div elements are just little elements with stuff in, which usually have an id of some sort telling developers where it lies within a page. A lot of statistical analysis was done to look at the most commonly occurring id and class names in the internet and it was found that things like header, footer, nav and content were used everywhere. These id's (with the exception of content) were taken into the HTML5 specification.
The HTML5 header, footer and nav elements are more machine readable and unambiguous, but the browser won't necessarily do anything special with it. This would be suitable for screen readers, although none support this yet. Page scrapers would also be able to use these elements to create an automated sitemap.
Old browsers will treat any unknown tag as an inline element, to get around this use the following style rule.
header, footer {display:block;}
Unfortunately, IE below version 9 still needs training wheels to get it working with HTML5 elements. All that is needed is to use the below JavaScript rule to get things running. What happens is that IE will see the elements created in memory and register that they exist. Without this code it won't recognise them as elements.
document.createElement('header');
document.createElement('footer');
The content div is an exception to the rule here as it was not defined as a HTML5 element. Instead, it uses what Patrick called the "Scooby do algorithm". Basically, the first element that isn't in a header or nav element is used as the content element.
There has been a lot of work in improving forms in the HTML5 specification to make the web more interactive and Patrick took us few most of them. There are too many to mention here so if you want to know the full list then take a look at the HTML5 spec for designers. Essentially, they have taken the standard input element and extended it to allow for all sorts of different things, including a whole load of date and time entry types. There are also some extra attributes that allow for things like auto focusing and auto complete.
Form validation is also available with the elements, so creating a URL or email element will automatically load some simple form validation controls. You can also enter your own validation by using the patterns attribute and entering a regular expression. This only works in browsers that support this and Opera is apparently the most advanced browser in terms of the supported form fields.
Before going any further Patrick asked if we would like to continue with the talk after lunch, to which there was lots of agreement. So we all went off to get some lunch and about 30 minutes later he came back and continued his talk.
The multimedia part of HTML5 is often what is sold the most, and is often referred to as the cool stuff. The main three parts to this are the video, audio and canvas elements.
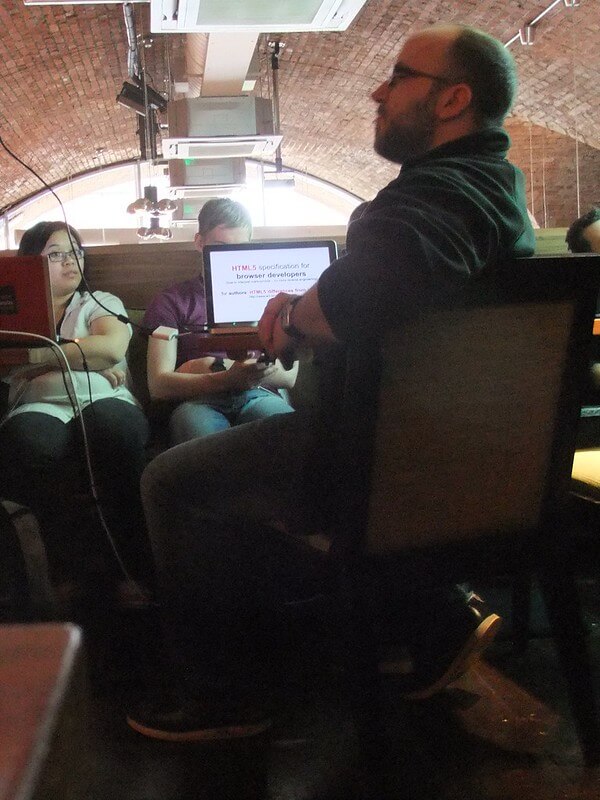
Picture taken by Lorna Mitchell.
The new video element was devised in order to replace the embedded flash element, which has almost become a cargo cult code phenomenon in itself. There are lots of advantages to the video element including keyboard accessibility as a default, the ability to control the player using JavaScript and the ability to skin the player with CSS. JavaScript can be used to control the player by calling functions like video.play() or video.pause().
A variety of video formats are also supported with the player. Patrick went through the three main ones of MP4/h.264, OGG/Theora and WebM/VP8 and gave an honest appraisal of where each format was in terms of licensing and support. There is quite a bit of debate going on at the moment into what is the best (in terms of technology and licensing) format to use is, and we were given a little insight into that. It is also possible to allow for multiple sources so that if one browser doesn't support that format another can be selected from the list. For legacy support a flash element can be embedded into the video element. The video element will be ignored if the browser doesn't understand it and use the object instead.
The audio element is similar to the video element in many respects. In fact, the same sort of debate with video formats is going on between browser vendors and the HTML5 spec writers. The main push of the format at the moment is in terms of MP3 vs Ogg Vorbis. Patrick also pointed out a few attributes for the audio element, including an autoplay feature. He said that this was being strongly debated at the moment, but that Opera would be looking at having a user definable setting to override this.
Finally, Patrick looked at the canvas element, which can be best described as a scriptable image. It doesn't do anything itself, but allows scripts to throw pixels at it to draw rectangles or whatever is needed. It is also possible to load images into the element. Patrick looked at lots of different examples of neat things that people were doing with this new element. Some of these were experiments and weren't really useful, but others included graphs, games and even image processing.
One of the benefits of using a canvas to render graphs is that you effectively pass the processing power needed to render the graph over to the client machine. Of course, at the moment not everyone can use HTML5 yet so legacy support means that you would need to create the graphs anyway. One of the disadvantages to using the canvas element is that they are really not accessible. It will, however, work on most new devices and browsers so support is becoming quite common.
This talk could have gone on for hours, in fact Patrick sped through his final 10-15 slides in about 20 seconds due to time constraints. But and I'm sure that we would all have sat there in silence listening intently to it. Patrick was a capable speaker, who gave a pragmatic approach to the new technology. He made it clear that older browsers and smartphones might not be able to support everything yet, but gave a good picture of what people were trying to do with HTML5. This talk was a great introduction to the new technology and I definitely feel more inclined to give the new spec a try now.
Even though he was from Opera, and he did use Opera in his demos and talk about what Opera were doing in this field, it wasn't a sales pitch. I'm pretty sure he didn't even set out with that in mind, which made the audience of open source developers and advocates warm to him a bit. I hear that Patrick lives locally so it would be great if we could get him back for another talk at one of our PHPNW meetups.
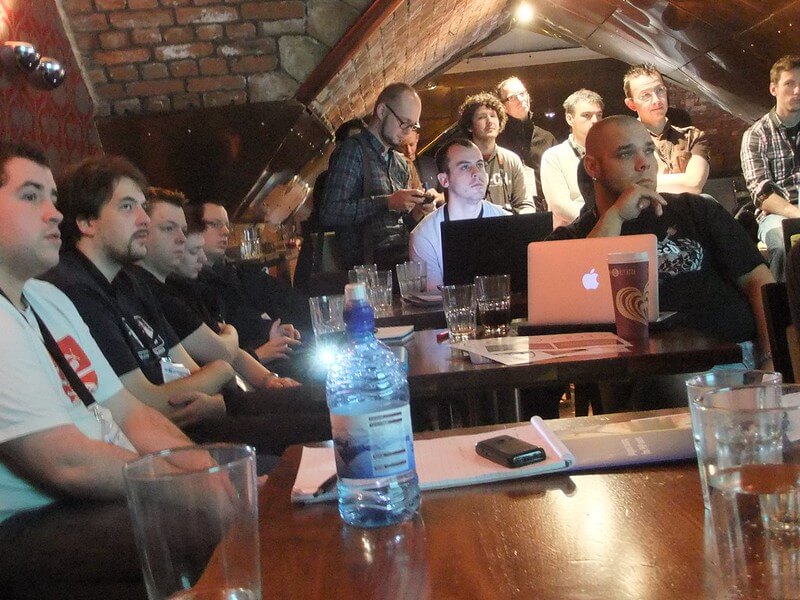
Picture taken by Lorna Mitchell.
Automated Release Procedures for the Web / DB Versioning and Deployment Sebastian Marek
This was a group discussion about the procedures that developers have for deploying both live code and also how they integrate changes to their databases during development. Which is a large and complicated topic that many developers have tried to solve in the past. The main course of action that seemed to come out of this group was to create upgrate patches that are run on the database every time there is a change. These patches can be run in order to bring an old version of the database up to the current version. The latest version of the database is always kept so that new developers can set up a new system quickly.
Of course, the ideal situation is to create your database in such a way that you don't need to change it later, but this almost never happens.
Someone in the group mentioned the subject of unit tests with different database versions, and someone else said that if a unit test includes a connection to a database then it isn't a unit test. I am quite new to unit testing, but I thought part of the idea was to test as much of the applications as possible, including the database interaction. I have recently heard of parts of PHPUnit called DbUnit which allow for testing of the database so I must assume that it is a common practice.
Much of the discussion centred around problems and solutions that I have heard before, but it was interesting to see other people's experiences in this field.
Setting up Jenkins CI for PHP Projects
Sebastian Bergmann and Volker Dusch
This was an introduction by Sebastian and Volker to the open source continuous integration environment called Jenkins; until very recently called Hudson. It was compared to other tools like PHPUndercontrol and most agreed that Jenkins was far easier to install and setup. I have tried to get PHPUndercontrol working in the past, but had eventually given up, so I could appreciate the sentiment here. The other complaint about PHPUndercontrol was that when it breaks, it breaks very hard and it is difficult to get it running again or your data back.
Jenkins allows lots of tools work together to provide a single continuous integration experience and so there is a lot of different XML files being passed around internally. A site that Sebastian has setup called jenkins-php.org provides lots of useful information on how to go from a installed copy to a full working version along with full PHP support.
To get Jenkins go to the main site at jenkins-ci.org and follow the instillation instructions there. As previously discussed Jenkins used to be called Hudson. It was forked from the Hudson project as it was part of Sun Microsystems when Oracle took them over.
Using Sebastian's site at jenkins-php.org you can install Jenkins and then copy and paste lines of code to run. You can do the configuration through the web interface, although it doesn't restart Jenkins so the changes won't take straight away.
One tip that Sebastian gave was to get the 'green ball' project to make the default blue balls that give indication of what is going on into green, which makes it easier to see what is going on.
The make file (eg, ant or phing) that runs and produces all of the usable xml files and will create all of the needed directories that are used. The rest of configuration is then interface based.
The weather icon that gave an indication of how healthy the project is seemed like quite a good indication of how things are going. Sebastian said that with legacy projects it will have a lot of violations, and working on the code helps you see if you have added any new violations, but you can also look at this icon to see if you have improved the project health at all.
Someone during this session said that these sort of systems act as information radiators, which I thought was a brilliant term. The talk was a great introduction to Jenkins and it has given me the confidence and drive to try it out for myself. The only problem I see if that I write a lot of Drupal code and so these systems are probably not well suited for that. I will have to experiment and see what I can find.
Conclusion
Overall the entire event was well planned and thoroughly enjoyable. Most of the talks were great, but it was also great talking to lots of interesting people about all sorts of things including the best virtual host to go for, bad coding standards, bad clients, the state of computer science education in the UK, being a sys admin, and even a quick discussion about Moodle. I think I have concentrated on writing about the talks in this review, but I wanted to stress the importance of the hallway tracks. Most of the event consisted of long coffee breaks and short talks, which means there was a lot of time to talk.
The turn out was impressive considering that many of the participants came from Germany and that the PHPUK 2011 conference was the Friday after the event. I would have expected the turn out to be low in favour of that event, but many PHP developers from the North West decided to attend the unconference in favour of PHPUK 2011. There is already talk about organising the next PHP Unconference Europe and I will be looking forward to it.
For more information on the PHP Unconference Europe have a look at the following links to the official sites:
Read Part 1 of this post here.
I would like to close on a little note about the venue for the weekend, which was the Pitcher and Piano, in Deansgate Locks in Manchester. You can probably tell from some of the comments above that it was a little bit noisy, but that doesn't really show just how inconsiderate they were towards us. Members of the public they can't control, so I'm not really complaining about that. What I am complaining about was the general disorganisation of the place and their (seemingly fanatical) insistence that there be some sort of music on all the time. It seemed like every half hour or so someone on the staff would turn on the music and we would have to go and tell them to turn it off again. There was one occasion where we were all stood in a large group downstairs, at 9am, and the music got turned on so loud that people literally couldn't hear each other speak. It was soon turned down, but what possessed them to turn it on I can only guess at.
What really took the biscuit and annoyed everybody was that although they insisted that the venue's wireless connection could cope with 100+ devices it clearly couldn't. At 9am on Saturday, when everyone got their laptops, netbooks, iPads or whatever out the first thing the network did was crash. In fact, it crashed every few minutes and there was a common theme of people getting very annoyed because their internet had gone again. This is a big pain for people who spend a *lot* of time on the internet, and it also made life a little bit more difficult for the speakers as some of them went onto the internet to show some things off.
The food served was good, and there was plenty of it, but I feel sorry for those people who are vegetarians. The vegetarian lasagne and wilted lettuce didn't look all that nice.
It's a shame that such a brilliant weekend was almost spoilt by the venue, and I understand that there was a relatively last minute change of venue so I'm not complaining about the organisers at all. But if you were thinking of holding an event in Manchester, especially if that event is in any way tech related, then I would urge you not to select the Pitcher and Piano.
Add new comment